We have only used the primary data type like int, char, double, etc. These are very useful but they can store only one value at any given time. Therefore, it can be used to handle only a limited amount of data. In many time, we need a strong data type which can store or handle a large volume of data in terms of reading, processing, and printing. C supports a derived data type known as an array that can be used for such works.
The array is a fixed-size sequenced collection of elements of the same data type under a single name. The ability to use a single name to represent a collection of elements and to refer to an element by specifying the element number enables us to develop a concise and efficient program.
We can use arrays to represent not only simple lists of values but also tables of data in two, three, or more dimensions. In this tutorial, we introduce the concept of an array and discuss how to use it to create the following type of arrays:-
- One-dimensional arrays
- Two-dimensional arrays or Multi-dimensional arrays
Declaration of one-D arrays
Like any other variable, Arrays also must be declared before they are used so that compiler can allocate memory. A general form of array declaration is:-
1 |
type_name variable_name [size] ; |
The type_name specifies the data type of element contained in arrays such as int, char, or float and the size indicates the number of elements stored inside the array.
For example:-
1 |
int class[100]; |
NOTE: The size field within square brackets [ ], representing the number of elements or items inside the array, must be a constant expression.
Initialization of One-D array
After declaration, its elements must be initialized otherwise it contains ‘garbage value’.The array can be initialized in two ways:-
- At Compile time
- At Run time
At Compile time
At compile time we initialize the elements of arrays in the same way as the ordinary variables when they are declared.A general form is:-
1 |
type_name array_name[size] = {list of elements} ; |
* elements are separated with help of commas.
For example:-
int a[10] = { 1, 2, 3, 4, 5, 6, 7, 8, 9, 0};
If the number of value in list is less than the number of element, then the remaining elements are be set to zero automatically.for example:-
At Run time
An array can be explicitly initialized at run time. This approach is usually applied for initialized large arrays with the help of a loop. For example:-
1 2 3 4 5 6 7 8 9 10 |
---------------------- ---------------------- for(i=0; i<5; i++) /* counter(i) help to increase index value */ { scanf("%d", &a[i] ); } ---------------------- ---------------------- |
We may use any loop like while,for,do-while for that purpose.Loop only works to change or increase the index value of array.
C Code Example
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
#include <stdio.h> void main() { int array[5],i; for(i=0;i<5;i++) { printf("\n Enter a integar: "); scanf("%d", &array[i] ); } printf("\n Entered array is:- "); for(i=0;i<5;i++) { printf(" %d",array[i]); } } |
Two-dimensional arrays or Multi-dimensional arrays
C allows arrays of two or more dimensions. The exact limit is determined by the compiler. we have discussed the array variable that can store a list of elements.There could be situations where a table of values will have to be stored. For example:-
The general form for two-dimensional arrays:-
Matrix represents a two-dimensional array of 3 per 3 elements of type int
1 |
int a[3][3]; |
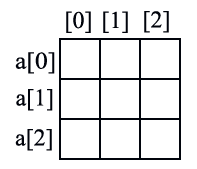
Multi-dimensional arrays are not limited to two indices (i.e, two dimensions arrays, three dimensions). They can contain as many indices as needed. A general form for multi-dimensional arrays is:-
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
#include <stdio.h> void main() { int array[3][3],i,j; for(i=0;i<3;i++) { for(j=0;j<3;j++) { printf("\n Enter a integar: "); scanf("%d", &array[i][j] ); } } printf("\n Entered Matrix is:-\n "); for(i=0;i<3;i++) { for(j=0;j<3;j++) { printf(" %d",array[i][j]); } printf("\n"); } } |