Like any other language, C has its own vocabulary and grammar. In this tutorial, we will discuss the concept of a variable and its types as they relative to the C programming language.
C Tokens
In a passage of text, individual words and punctuation marks are called tokens. Similarly, in a C program, the smallest individual units are known as C Tokens. C has six types of Token
- Keywords
- Identifier
- constants
- Strings
- special symbol
- Operators
Keywords
In C Programming, keywords are said to be reserved words whose has a fixed meaning and these meaning cannot be changed. But it tells the compiler what to do. Keywords are always in lowercase letters. It cannot be used as an identifier.
Some common keywords are:-
- break
- if
- else
- while
- for
- do
- goto
- jump
- void
Identifier
Identifier refers to the name it may be the name of variable, function, and arrays. These are user-defined names and consist of a sequence of letters and digits. underscore character is also permitted as an identifier. Spaces, punctuation marks, and symbols cannot be part of an identifier.
For example:-
id a Name _1
The C language is a “case sensitive” language. That means that an identifier written in capital letters is not equivalent to another one with the same name but written in small letters.
Variables
A variable is a data name that may be used to store a data value. It may take different values at different times during execution.
C Code Example
1 2 3 4 5 6 7 |
#include <stdio.h> void main() { int a,b; a=5; b=2; a = a + 1; b = b - 1; } |
Obviously, this is a very simple example, since we have only used two small integer values, but consider that computers can store millions of numbers like these at the same time.
Data Type
C language is rich in its data type. It is the storage format to store data. The variety of data types available allows the programmer to select the type appropriate to the needs of the application as well as the machine.
C Support three type of data types:-
- Primary (or fundamental) data type
- Derived data type
- User-defined data type
Primary (or fundamental) data types
In this tutorial, primary data type and their extensions are discussed. Derived and user-defined datatype are discussed when array, pointer, functions are encountered.
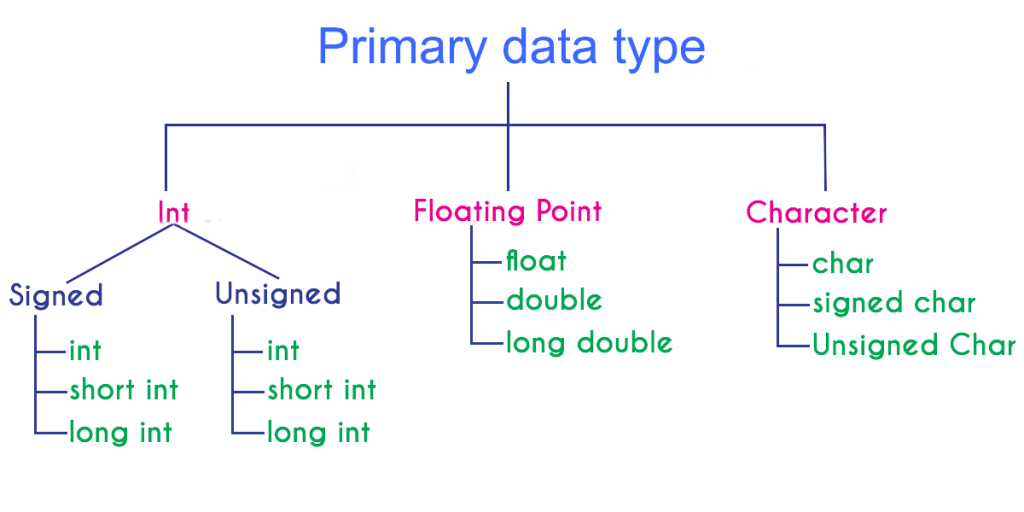
Integer type
An integer is a whole number with a range of values supported by the particular compiler. Generally, integers occupy one word of storage, and since the word sizes of compiler vary(typically 16 bit,32, and 64 bit). If we use 16-bit word length then the size of an integer value is limited to range -32768 to +32767.
In order to provide some control over the range of number and storage space, C has two classes of integer, Signed and unsigned.
Floating-point types
Floating point (or real) numbers are stored in 32 bits (on all 16 bit and 32-bit compiler) with 6 digits of precision. Floating-point numbers are defined in C by the keyword float. when the accuracy provided by a float number is not fulfilled, double can be used to define the number. A double data type number uses 64 bits giving a precision of 14 digits. To extend the precision further, we may use long.
Character types
The character can be defined as Character type data. The qualifier Signed or Unsigned may be explicitly applied to char While Signed char have a value from -128 to 127, Unsigned chars have value 0 to 255.
Declaration of Variables
After designing Variable name, we must declare them to the compiler, Declaration does two things:-
- It tells the compiler what the variable is.
- It specifies what type of data the variable will hold that variable.
* The declarations of variables must be before they are used in the program.
Primary type declaration
A variable can be used to store a value of any data type. That is, the name has nothing to do with its type.The syntax is as follow:-
data-type var-name,name-2;
Var-name,name-22 are the name of the variable which are seprated by commas.A declaration must end with a semicolon.For example,valid declaration are:-
- int Rollno;
- char a,b;
- double number, marks;
* int, char and double are the keyword which represents data type.
C Code Example
1 2 3 4 5 6 7 |
#include <stdio.h> void main() { int Rollno; scanf("%d",&Rollno); printf("Rollno=%d",Rollno); } |
Assigning a value to variables
values can be assigned to variables in 2 ways.
- Using the assignment operator.
- Reading data from Keyboard
Using assignment operator
Values can be assigned to a variable using the assignment operator (=) as follow:-
Variable-name=constant-value;
For Example:-
- Rollno = 15;
- number =1833 ;
- marks = 98 ;
It also possible to assign a value to a variable at the time of variable is declared. This takes the following form
data-type Variable-name=constant-value;
For Example:-
- int Rollno = 16023;
- int number =62 ;
This process of giving initial values to a variable is called initialization. C permits the initialization of more than one variable in one statement using multiple assignment operators.For example:-
- int a,b,c;
- a = b = c = 0;
C Code Example
1 2 3 4 5 6 7 |
#include <stdio.h> void main() { int a,b,c=9; a=b=4; printf("Value of a,b,c is= %d %d %d",a,b,c); } |
Reading data from Keyboard
Another way is through the keyboard using scanf function.It is a general input function available in C. and is a very similar concept to the printf function. We discuss scanf function later.
For example
C Code Example
1 2 3 4 5 6 7 8 |
#include <stdio.h> void main() { int a; printf("enter a"); scanf("%d",&a); } |